In the ever-evolving world of software development, understanding different programming paradigms is crucial to mastering the craft. One such paradigm that has significantly shaped modern programming is Object-Oriented Programming (OOP). If you’ve ever wondered why certain software feels more intuitive, scalable, or easy to maintain, it’s likely because of OOP’s influence. But what exactly is OOP, and why has it become such a fundamental approach in software design?
From Data to Objects: The Core Concept of OOP
At its heart, Object-Oriented Programming (OOP) is a model that organizes software around objects rather than actions and data rather than logic. Imagine software as a collection of interacting entities, each with its own responsibilities and characteristics. These entities, known as objects, are the core components of OOP. They represent real-world or abstract items that the program needs to manipulate, such as a customer in a banking application or a button in a user interface.
The Shift in Perspective: Why Objects Over Logic?
Traditional programming methods often focus on the logical sequence of operations required to achieve a task. However, OOP shifts this focus to the objects that need to be manipulated to perform these tasks. This shift is particularly beneficial in large, complex, and actively maintained software systems. For instance, OOP is ideal for manufacturing systems, where each machine or process can be modeled as an object, making the system more manageable and adaptable to change.
Building Blocks of OOP: Classes, Objects, Methods, and Attributes
To truly grasp OOP, it’s essential to understand its basic building blocks:
Classes: Think of a class as a blueprint. It defines the structure and behavior of objects but is not an object itself. For example, you might have a class called "Car" that defines attributes like color, model, and speed, along with methods that describe what a car can do, like accelerate or brake.
Objects: An object is an instance of a class. If the class is the blueprint, the object is the actual car created from that blueprint. Each object can have different attribute values but will follow the structure and behavior defined by its class.
Methods: These are functions defined within a class that describe the actions an object can perform. For example, a "Car" class might have methods like "startEngine" or "stopEngine."
Attributes: Attributes are the characteristics or properties of an object, stored as data within the object. In our "Car" example, attributes might include the car’s color, make, and current speed.
Encapsulation: Keeping It All Together
One of the foundational principles of OOP is encapsulation. This principle states that all important information within an object is contained and hidden from the outside world, exposing only what is necessary through well-defined interfaces. Encapsulation not only protects the integrity of the object’s data but also simplifies the interaction with the object. By hiding the internal workings, developers can use objects without needing to understand the complex details of their implementation.
Abstraction: Simplifying Complexity
Closely related to encapsulation is the concept of abstraction. Abstraction in OOP means that objects only reveal the necessary parts of their internal mechanisms to other objects. This allows for a more straightforward interface and hides the unnecessary details. For instance, when you drive a car, you don’t need to understand the intricate details of the engine. You only need to know how to operate the steering wheel, pedals, and gear shift. Similarly, in OOP, objects expose only the relevant functionalities, making the system easier to understand and extend.
Inheritance: Reusing Code and Building Hierarchies
Inheritance is another key feature of OOP that promotes code reusability and the creation of hierarchical relationships between objects. When a class inherits from another, it gains access to the attributes and methods of the parent class while allowing for the addition or modification of features. This reduces redundancy and fosters a more organized and logical structure in the codebase. For example, if you have a "Vehicle" class, you could create a "Car" class that inherits from "Vehicle," adding specific attributes like "number of doors" while reusing common attributes like "engine type."
Polymorphism: Flexibility in Action
Polymorphism allows objects to be treated as instances of their parent class, even when they are not. This means that different classes can be treated through the same interface, reducing the complexity of the code. For example, both "Car" and "Bike" could inherit from the "Vehicle" class. Despite their differences, they can be interacted with through a common "Vehicle" interface, with each class implementing its unique behavior for the methods defined in "Vehicle." This flexibility is one of the reasons OOP is favored in large, dynamic applications.
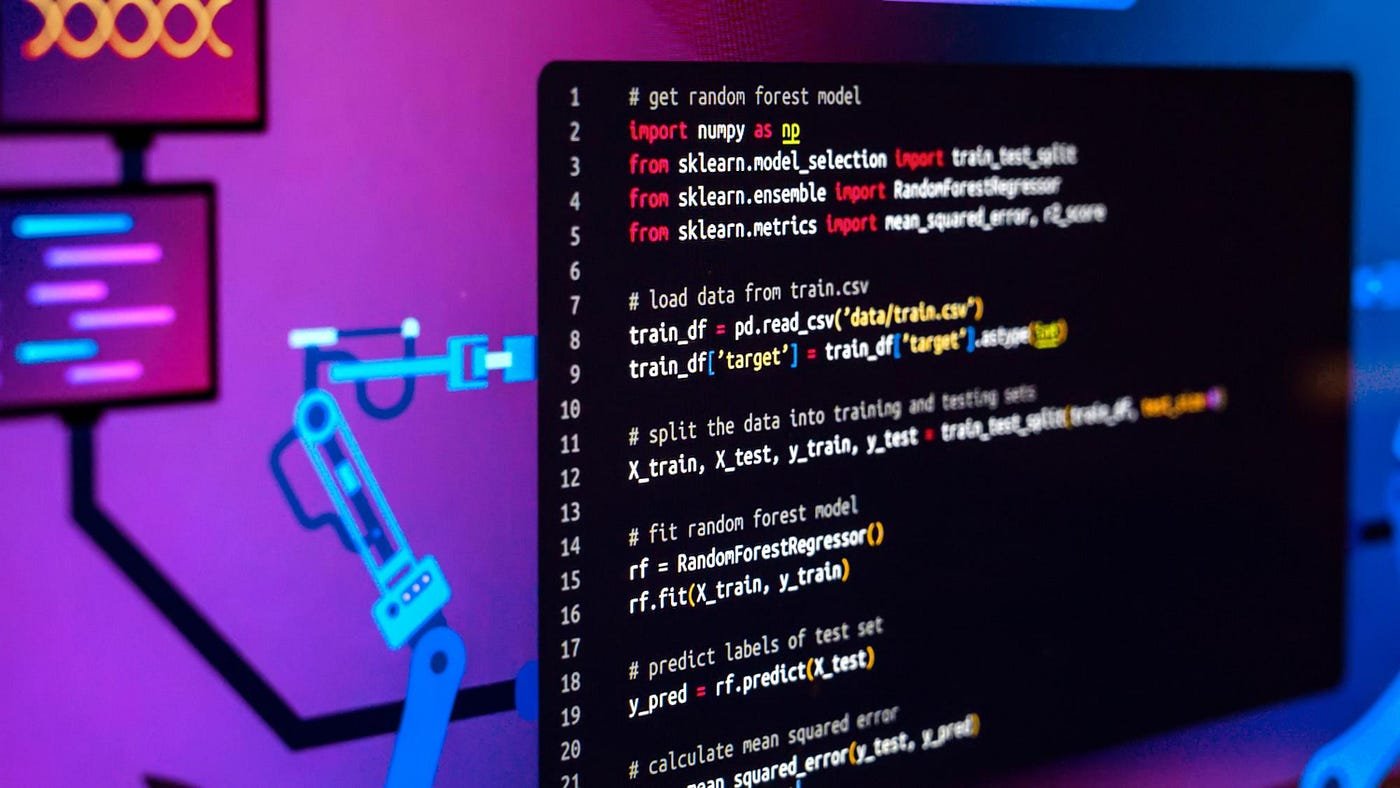
A Look at OOP Languages: Which Ones Should You Know?
While the concept of OOP can be applied in many programming languages, some are specifically designed to leverage OOP principles more effectively.
Pure OOP Languages: These languages treat everything as an object. Examples include Ruby, Scala, JADE, and Emerald. These languages are designed from the ground up with OOP principles in mind.
Languages Primarily Designed for OOP: Languages like Java and Python are not pure OOP languages but are heavily oriented toward OOP. They allow a mix of procedural and object-oriented programming, making them versatile for different types of projects.
Other OOP-Compatible Languages: Languages such as Visual Basic .NET, PHP, and JavaScript also support OOP. They might not be exclusively OOP, but they provide the tools necessary to implement OOP concepts effectively.
More News: best 10 latest tech gadgets and reviews
The Perks of OOP: Why Developers Love It
So, why is OOP so widely adopted in software development? The answer lies in its numerous advantages:
Modularity: Encapsulation makes objects self-contained, making it easier to troubleshoot and collaborate on development projects.
Reusability: Inheritance allows developers to reuse existing code, reducing duplication and saving time.
Productivity: By reusing code and utilizing existing libraries, developers can create new programs more quickly.
Scalability: OOP’s modularity makes it easier to scale applications, as new features can be added with minimal impact on existing code.
Security: Encapsulation and abstraction protect data, making software more secure and less prone to errors.
Flexibility: Polymorphism allows for more flexible and dynamic code, enabling developers to adapt to changing requirements easily.
Maintainability: OOP code is easier to maintain and update, thanks to its clear structure and modular design.
Cost-Effectiveness: All these benefits translate into lower development costs, as less time is spent on redundant tasks, and more focus is placed on innovation and quality.
The Flip Side: Criticisms of OOP
Despite its many advantages, OOP is not without its critics. Some developers argue that OOP can sometimes lead to overly complex code, especially when the object hierarchy becomes too deep or convoluted. Others point out that OOP tends to emphasize data over algorithms, which may not always be the best approach for every problem.
Additionally, the inheritance model, while powerful, can lead to issues such as "fragile base class" problems, where changes to a parent class inadvertently affect all its subclasses, potentially introducing bugs. Moreover, understanding an object in isolation is often easier than understanding how it interacts with the entire system, leading to potential confusion.
Alternatives to OOP: Exploring Other Paradigms
Given these criticisms, it’s worth noting that OOP is not the only programming paradigm available. Developers have other options, depending on the nature of the problem they are trying to solve:
Functional Programming: Languages like Erlang and Scala focus on mathematical functions and avoid changing states, making them ideal for telecommunications and fault-tolerant systems.
Structured or Modular Programming: This approach, used in languages like PHP and C#, focuses on breaking down programs into smaller, manageable modules.
Imperative Programming: Languages like C++ and Java emphasize the use of statements to change a program’s state, focusing more on the "how" rather than the "what."
Declarative Programming: Languages like Prolog and Lisp describe what the program should accomplish without specifying how, often used in logical programming and databases.
Is OOP Right for Your Project?
Whether OOP is the right approach for your project depends on various factors, including the complexity of the software, the team’s familiarity with OOP, and the specific requirements of the task at hand. While OOP offers numerous benefits, it’s not always the best fit, especially for simpler projects where other paradigms might be more efficient.
Read More: WWW: A Journey from Tim Berners-Lee’s Vision to the Digital Future
Conclusion: Harnessing the Power of OOP
Object-Oriented Programming has undoubtedly changed the way developers approach software design, making it possible to create more modular, reusable, and scalable applications. Whether you're building a simple app or a complex system, understanding the strengths and limitations of OOP can help you decide whether it's the best approach for your project. If you're new to OOP, start by experimenting with simple projects to get a feel for its concepts and benefits. Over time, you'll be able to leverage OOP to create more robust, flexible, and maintainable software solutions.
Other Popular Technology Post:
Samsung Galaxy S25 | Realme GT7 Pro | ChatGPT | Snapdragon 8 Elite Smartphones | AI Tools And Software | What Is E-commerce | Radio Frequency | Paid Time Off Policy | BIOS Update | Logic Gates | QA Quality Assurance | Fiber Optics Technology | What Is Excel | Amazon Diwali Sale 2024 | Meta AI's Chat Memory | World Wide Web
Explore other popular Posts:
Blog | News | Entertainment | Education | Sports |
Technology | Cryptocurrency | Stock | Home | Sitemap